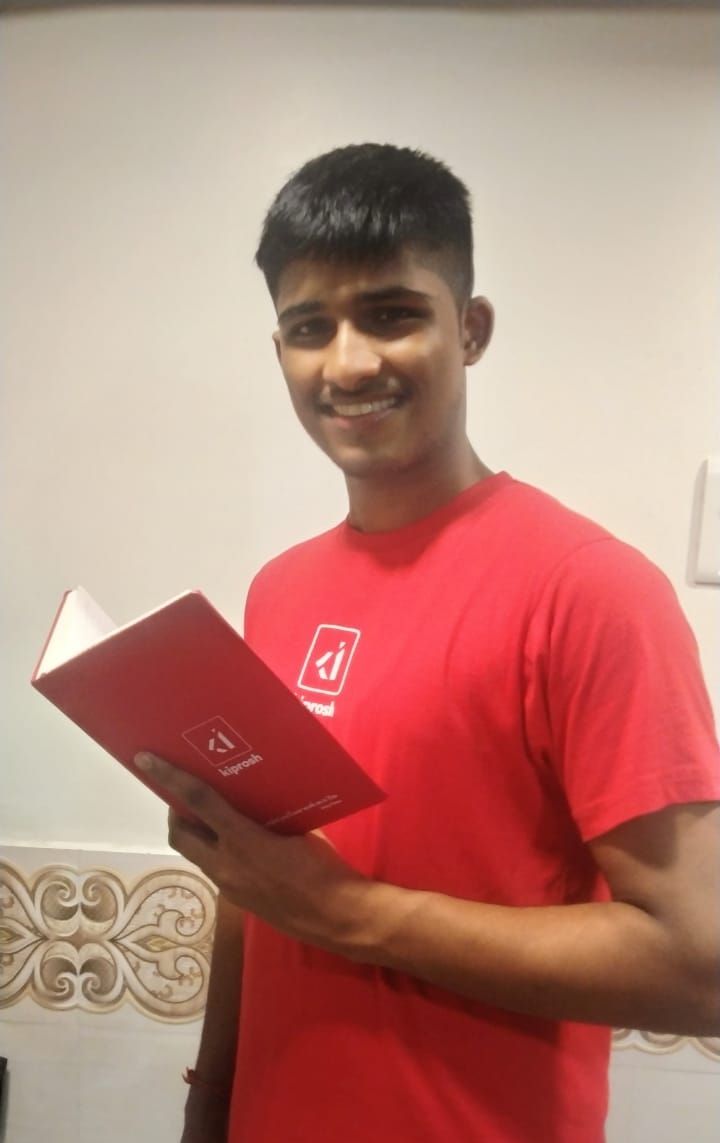
Ruby 3.2.0 enhances Regexp performance and security with ReDoS protections
What is ReDoS? Regular expression Denial of Service (ReDoS) is a security vulnerability that can occur in a regular expression (regex) when the regex is applied to a long string. This attack is designed to make a system or network unavailable to its intended users. An example occurrence of a ReDoS Imagine that a website has a form that accepts user input and uses a regex to validate the input. The regex is designed to only allow alphanumeric characters in the input, so it looks like this: /^[a-zA-Z0-9]+$/. An attacker could potentially craft a string of input that consists of
Rails 7.1 adds the --parent option to the Job Generator
Rails 7.1 adds the --parent option using which we can create a job that inherits from the superclass. Let's learn how to use it.
Turbo - The heart of Hotwire
Turbo provides the most basic building blocks for building Hotwire application. In this article, let's learn more about its features.
Rails 7 introduces partial_inserts config for ActiveRecord
Rails 7 adds the partial_inserts config to config.active_record that is used to control partial writes when creating a new record. config.active_record.partial_inserts is a boolean value and controls whether or not partial writes are used when creating new records (i.e. whether inserts only set attributes that are different from the default). Configuring config.active_record.partial_inserts In a newly created Rails 7.0 application, config.load_defaults 7.0 is set by default in application.rb. The default value of partial_inserts for config.load_defaults 7.0 is false and for
Speeding up Rails 7's Controller Actions using ActiveRecord's #load_async
Most of the time in a web application, a single API request consists of multiple database queries. For example: class DashboardsController < ApplicationController def dashboard @users = User.some_complex_scope.load_async @products = Product.some_complex_scope.load_async @library_files = LibraryFile.some_complex_scope.load_async end end Quoting snippet from load_async PR description from rails repository. The queries are executed synchronously, which mostly isn’t a huge concern. But, as the database grows larger in size, the response time of requests is getting longer. A significant part of the query time is often just I/O waits.
How to prevent race condition in Ruby on Rails applications?
Race conditions are always surprising, which can occur in production and are difficult to reproduce. They can cause duplication of records in the database. Most of the time the locking mechanism is taken care of by the Rails framework. Users don't have to manage it; especially optimistic locking using the lock_version column. In case of transactions and race around conditions, we can prevent these issues with Pessimistic Locking in ActiveRecord. It locks a record immediately as soon as the lock is requested(uses database row-level locking). Race conditions happen when two users read and update a record at the
Adding Two-Factor Authentication(2FA) to ActiveAdmin auth in a Ruby on Rails web application
To enhance the security of a web application having a user authentication workflow, we use a security method called 2FA. It is also known as Two Factor Authentication(type of Multi-Factor Authentication). In this blog post, we will see how to implement email-based 2FA in ActiveAdmin auth of a Ruby on Rails application. In the email-based 2FA approach, when logging in with an email and password, an OTP will be sent on a registered email address. Upon entering the OTP, it will successfully authenticate and the session will be started. Also, we will see the following additional functionality and customizations