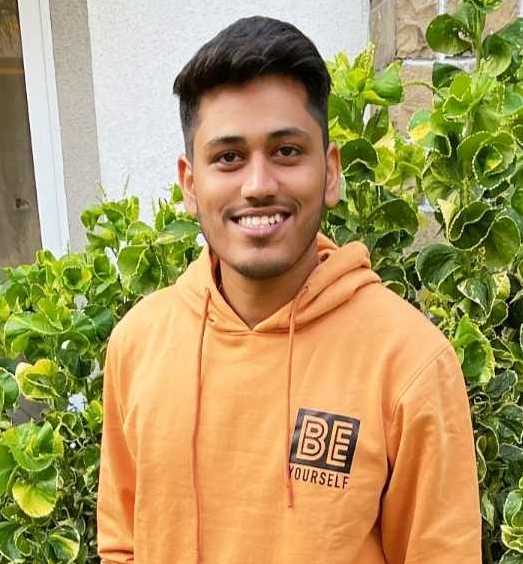
All about "Data" Simple Immutable Value Objects in Ruby 3.2
In Ruby 3.2, a new class Data was introduced as a way to define simple immutable value objects. A value object is a type of object that represents a value in a program, such as a point in 2D space or a date. The main advantage of value objects is that they are easy to understand, simple to use, and can improve the readability and maintainability of code. The proposal to add Data class was accepted by Matz on the Ruby forum here. How does it work?Using the newly defined class Data we can create a simple immutable
Rails 7.1 supports password challenge via has_secure_password
Rails provides the has_secure_password method, which makes it gloriously easy to implement authentication in our application. But we often need an extra layer of verification before allowing users to update certain fields. For e.g. Users must provide their “old” password when updating their email/password fields. Before Rails 7.1To implement this, we must manually add and validate the current_password accessor: # app/models/user.rb class User < ActiveRecord::Base has_secure_password attr_accessor :current_password end# app/controllers/passwords_controller.rb class PasswordsController < ApplicationController def update password_challenge = password_params.delete(:current_password)
Ruby 3 adds Scheduler Interface for Fibers
Ruby adds Fiber SchedulerInterface to support non-blocking fiber. Splitting long operations into fiber hooks and Scheduler will manage it for us.
Rails 7 introduces ComparisonValidator to compare data with validation
Rails 7 adds ComparisonValidator to compare and validate numeric, date and string values. It provides comparisons options that accept value, procs, or symbol.
Deep dive into ActiveRecord .scoping method in Rails
In Rails, multiple scopes can be created and chained together. What if we wish to apply a specific scope to a group of queries? Consider the following scenario: we have Post and Comment models and we want to perform few operations on public posts. # app/models/post.rb class Post < ActiveRecord::Base scope :public, -> { where(private: false) } endLoading development environment (Rails 7.0.0.alpha2) 3.0.0 :001 > Post.public.update_all(body: 'public post') Post Update All (4.1ms) UPDATE "posts" SET "body" = ? WHERE "posts"."private" = ? [["body", "public post"], ["private", 0]] 3.0.0