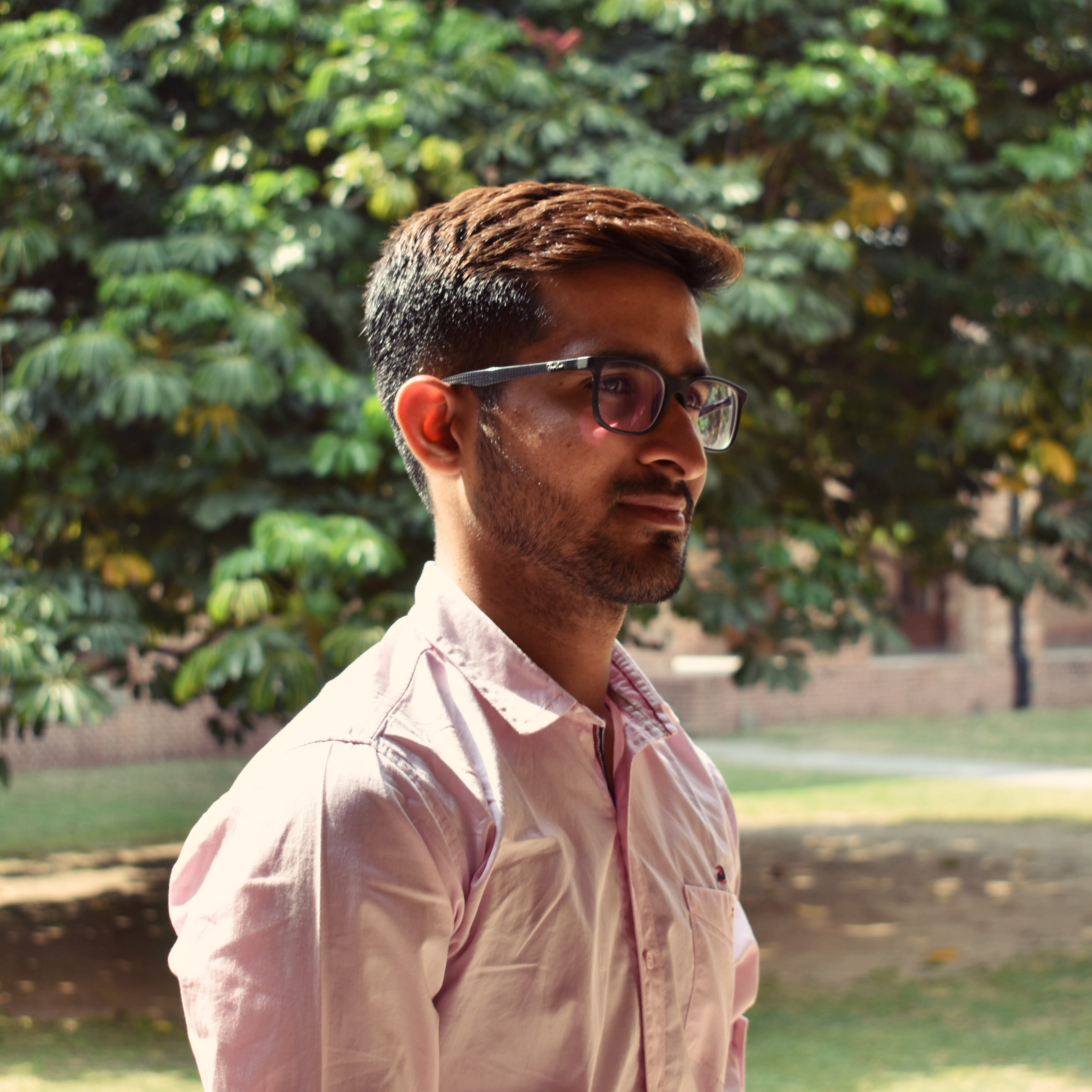
JavaScript Module design pattern
JavaScript modules are the most used design pattern to keep your code separate from your components which provides loose coupling to your code. Modules are JavaScript classes, which provides protection of states and behaviours from being accessed by other classes. The module pattern allows for public and private access levels. This is how the code looks like: (function() { // declare private variables and/or functions return { // declare public variables and/or functions } })(); Here we have to instantiate the private variables and functions before returning our object. Code outside of our function is unable to access these private variables since it is
JavaScript callBack/Higher-order functions
Functions are objects in JavaScript, so you can store them in variables, pass it as an argument to another function and also you can return it from the function. Yes, function returning another function. CallBack functions are derived from functional programming and is also covered in advanced JavaScript topics but here we'll see that it is quite easy to implement. What is a CallBack or Higher-order function? It is a function which is passed to another function as parameter and is called inside that another function, therefore know as callBack function. Example of callBack function in jQuery: $("#btn"
Build MySQL Docker container image
In this article I'm gonna show you how to build MySQL Docker container image. This article assumes that you've some basic knowledge about Docker. To run MySQL on Docker we would need to do the following Install Docker engine on your computer Download MySQL image from Docker Hub And run the container First, get the MySQL image docker pull mysql You'll need to set up your credentials and specify path for MySQL docker run -d --name=mysql1 -e MYSQL_ROOT_PASSWORD=’mypassword’ -v /storage/mysql1/mysql-datadir:/var/lib/mysql mysql docker run -d --name=mysql2 -e MYSQL_ROOT_PASSWORD=’mypassword’
Setting StatusBar manually for Cordova application using cordova-plugin-statusbar
We've couple of Android and IOS apps which was made using Cordova. After upgrading our cordova-ios and cordova-android versions we were facing some issues with native status-bar. The status-bar content and page content were getting mixed up, making user unable to perform any operations provided in the header. While looking for solution we found cordova plugin cordova-plugin-statusbar which provides some functions to customize the iOS and Android StatusBar. To use this plugin we need to add some lines to our config.xml file in cordova folder. First we need to add permission for cordova-plugin-statusbar to make changes to the status-bar.
Model level validations in Ember.js
We've couple of Ember apps which communicates to single rails API. We were working on making our API more robust for data related operations so we added couple of checks and validations which led us to a thought that it would be even better if we can add validations on our front-end Ember applications. But Ember.js framework doesn't provide validations for models. So we investigated further and we found couple of packages that we can use for model level validations. Ember-model-validator is the one of the most used package for model validations in Ember. It is really easy to
Force reloading specific record in Ember.
I was facing an issue while working on one of my Ember Apps. Issue was like, Ember data store was not updating the record after receiving the response from backend. So whenever user visit the page, it was showing the old data of that record from Ember-Data store. After some searching I found that Ember-data by default doesn't re-request data that is already present in Ember-data store. If parent route's model has loaded an array of objects then the child route that requires specific object will not make another request. In such case we can force reload the record. this.
The jQuery Mockjax Plugin for mocking or simulating ajax requests and responses
Currently I've been working on writing acceptance test cases for my project in which there were lot of scenarios where I'd to use mocking/stubbing for which I used MockJax plugin of jquery. So I'm sharing some examples which might be useful to someone. A simple mock request using mockjax plugin can be written as $.mockjax({ url: '/posts/1', responseTime: 500, responseText: { status: 'success', post: 'Mockjax Plugin for mocking or simulating ajax requests and responses' } }); Here we can specify the time it will take to get the response and the text which we'll be getting in the response. This
SideLoading, Active Model Serializer and Adapter and Links in Ember
Side Loading Ember Data supports “sideloading” of data; i.e., indicating that data should be retrieved (along with the primary data requested) so as to help multiple related HTTP requests. A common use case is sideloading associated models. For example, each Shop has many groceries, so we can include all related groceries in /shops response: GET /shops { "shops": [ { "id": "14", "groceries": ["98", "99", "112"] } ] } When the groceries association is accessed, Ember Data will issue: GET /groceries?ids[]=98&ids[]=99&ids[]=112 { "groceries&
Ember-Data library for Ember.js
Ember-Data is a library for managing model data in Ember.js applications. It provides many of the facilities you’d find in server-side object relational mappings (ORMs) like ActiveRecord, but is designed specifically for the unique environment of JavaScript in the browser. When an API is represented using Ember Data models, adapters and serializers, each association simply becomes a field name. This encapsulates the internal details of each association, thereby insulating the rest of your code from changes to the associations themselves. Routes and Models In Ember.js, the router is responsible for displaying templates, loading data, and otherwise setting
Integrating Sendgrid with your Application to receive Email related Events.
We've one Blogging application and whenever User post a new Article we wanted to show that to whom his/her post is delivered, who has opened a post or clicked.. events like that. So that User will know how many people are reading his/her post and also how often. We were already using services of Sendgrid. Now Sendgrid provides different APIs for different purposes. Some of them I'm listing below. SMTP API, WEB API, EVENT WEBHOOK, PARSE API. Now, We needed EVENT WEBHOOK API for what we wanted to achieve. Here is a link to EVENT WEBHOOK API. The