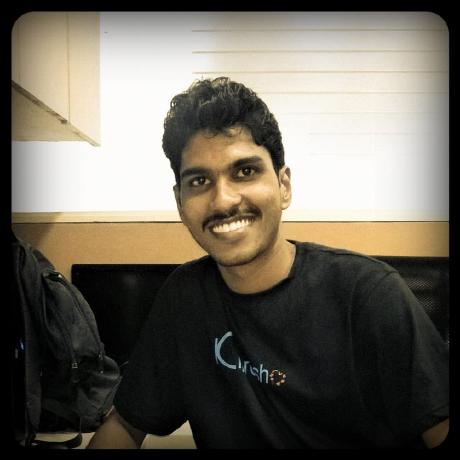
Sampat Badhe
I am a Rubyist and Node.js developer at Kiprosh.com. I enjoy building innovative applications and currently in love with TDD practice. I am practicing Agile and love to pair program.
14 posts
Kiprosh
is now part of
LawLytics
Sometimes you may want to standardize the data before saving it to the database. For example, downcasing emails, removing any leading and trailing whitespaces, and so on. Rails 7.1 adds ActiveRecord::Base::normalizes API, which allows you to normalize attribute values to a common format before saving them to the database. This can help improve the integrity and consistency of your data and make it simpler to query records based on attribute values. Before Rails 7.1 Normalizing it in before_validation or before_save callback class User < ActiveRecord::Base before_validation do self.name = name.downcase.titleize
Rails 7.1 adds the raise_on_assign_to_attr_readonly config to config.active_record that is used to raise ActiveRecord::ReadonlyAttributeError error on assignment to attr_readonly attributes. The previous behavior would allow assignment but silently not persist changes to the database. Configuring config.active_record.raise_on_assign_to_attr_readonly In a newly created Rails 7.1 application, config.load_defaults 7.1 is set by default in application.rb. The default value of raise_on_assign_to_attr_readonly for config.load_defaults 7.1 is true and for config.load_defaults < 7.1
An ActiveRecord query by default uses the SELECT * operator to select all the fields from the result set. The ActiveRecord::QueryMethods#select method allows you to select a subset of fields from the result set. Before Rails 7.1 ActiveRecord::QueryMethods#select and ActiveRecord::QueryMethods#reselect only accept strings, symbols, or raw SQL to define columns and aliases to select. When querying a single model, we can provide column names as an array of strings or symbols, or we can provide raw SQL. > Model.select(:field, :other_field) > # OR > Model.select('field', 'other_field') > # OR >
In Rails, Active Record provides batch processing for ActiveRecord::Relation with the in_batches method. In Rails 7.1 the implementation of in_batches has improved to give optimized results for whole table iterations. In this article, we will see how it has improved. Example User.in_batches(of: 3) do |relation| puts relation.to_sql end Before Rails 7.1 Loading development environment (Rails 7.0.4) 3.0.0 :001 > User.in_batches(of: 3) do |relation| 3.0.0 :002 > puts relation.to_sql 3.0.0 :003 > end User Pluck (0.4ms) SELECT
Rails 7.1 introduces a method authenticate_by, used with has_secure_password to prevent timing-based enumeration attacks.
Rails framework is famous for developers' happiness and making things simpler due to its magic, provided developers follow proper conventions. To extend this magic and to make things simple further, Rails 7 has introduced a change with this PR after which, inverse_of would be inferred automatically for model associations having scopes. In this article, we'll dive into understanding it with examples. Let's say we have a Project model with many assigned tasks. # app/models/project.rb class Project < ActiveRecord::Base has_many :tasks, -> { assigned } end # app/models/task.rb class Task < ActiveRecord::Base belongs_to
Rails 7 allows to fetch data from multiple databases for a has_many/has_one:through association using the disabled_joins option.
Authentication is one of the key aspects of many web applications. It is the process of identifying a person before granting them access to the application. It is very important that the authentication approach is secure and easy to use for all users. What is passwordless authentication? Passwordless authentication is a verification process that determines whether an individual is who they claim to be, without coercion. You do not require credentials to log in. All you need is an email address or phone number associated with an account and you will get a magic link or one-time password each time
In the previous article, we went through How NOT IN works with NULL values. We also learned how we can overcome this restriction. In this article, we will look at alternative ways to handle NULL values with SQL functions. So basically when we use != or NOT IN in query, it ignores the records with NULL values for fields. User.where("state != ?", 'active') SELECT * FROM users WHERE state != 'active' OR User.where("state NOT IN (?)", ['active']) SELECT * FROM users WHERE state NOT IN ('active') The above queries will consider records with state having a NOT NULL value,
Code reviews are important to deliver quality and well-crafted software. But what if the PR lingers in the review queue waiting for reviewers for an extended period? We faced a similar issue in one of our team where few stories were delayed due to pending reviews from the members. In this article, I would like to share about how we improved the Code and Functional Review process resulting in high quality and happiness. *This process was identified and implemented for one of the projects and every project could have minor customization based on the nature and requirement of the project.