Wistia is an embeddable player that is customizable and provides a rich interface to change player color, adding logos, choosing a thumbnail, etc. But can we get even better control by using external scripts that can change the player, are asynchronous, cached and are portable between projects? Yes! with Wistia plugins these features come alive and with no extra effort.
The Science of Wistia Plugins
Consider the Wistia plugin as an API that provides access to the player, the video, and the controls to an external script. It exposes the reference to the internal video object to customize it as required. The important property of this video is the Grid which provides shortcuts to DOM elements around the video, these are helpful in inserting, styling, and controlling new elements on the Player.
Creating a plugin
You can create Wistia plugin by using the syntax Wistia.plugin("pluginName", function)
The function will receive two parameters, the video object and any extra arguments passed to the plugin
Wistia.plugin("showVolume", (video, options) => {
video.bind("play", ()=>{
console.info(video.volume())
});
});
The 👆 Wistia plugin will log the volume of the video when played. More about what you can access with the video
object and events that can be handled is available in the list of player methods
Using a plugin
Using a plugin in Wistia is simple as pushing the config into the Video Handler window._wq
with a touch of "plugin"
key
window._wq = window._wq || [];
window._wq.push({ "j38ihh83m5": {
plugin: {
"showVolume": {
src: "./wistia-plugin.js"
}
}
}});
When video with id j38ihh83m5
is loaded, the plugin will be called. More about the Wistia video handlers is available in the player API
Plugin for Custom Controls
Let's create a plugin that will allow custom keyboard controls over any video that it is included in.
Key | Action |
---|---|
1 | Playback speed 1x |
2 | Playback Speed 2x |
a | Backwards 5 seconds |
d | Forward 5 seconds |
Into the code
- Html layout -
wistia-embed.html
<!DOCTYPE html>
<html>
<head>
<script
src="//fast.wistia.com/embed/medias/j38ihh83m5.jsonp"
async
></script>
<script src="//fast.wistia.com/assets/external/E-v1.js" async></script>
<script src="./src/wistia-player.js"></script>
</head>
<body>
<div
class="wistia_embed player1 wistia_async_j38ihh83m5"
style="height: 349px; width: 620px;"
>
</div>
</body>
</html>
2. Custom plugin - wistia-plugin.js
Wistia.plugin("keyboardControls", (video, options) => {
const { player } = options;
const keyDownHandler = (event) => {
const { key } = event;
switch (key) {
case "1":
video.playbackRate(1);
break;
case "2":
video.playbackRate(2);
break;
case "a":
video.time(video.time() - 5);
break;
case "d":
video.time(video.time() + 5);
break;
default:
break;
}
};
video.bind("play", () => {
player.addEventListener("keydown", keyDownHandler);
});
});
This plugin will make the video being played, respond to the key down events, and take actions.
3. Using the custom plugin - wistia-player.js
window.onload = ()=>{
const player = document.querySelector(".player1");
window._wq = window._wq || [];
window._wq.push({
j38ihh83m5: {
plugin: {
keyboardControls: {
player: player,
src: "./wistia-plugin.js"
}
}
}
})
};
That's it! the video now can be controlled using our custom controls. To include it in all of the videos, you can pass _all
instead of a video id. This plugin can be moved anywhere across projects and reused by including it.
Watch the demo on CodePen
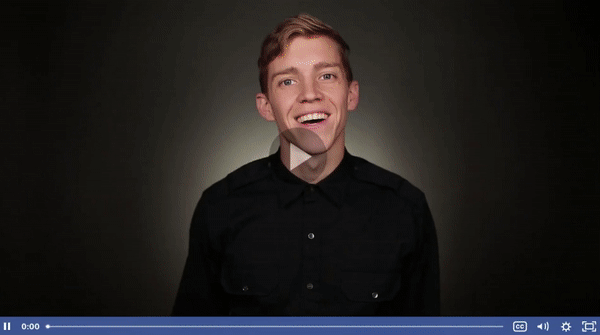
Read more about the plugins in the references and start customizing.