In today's fast-paced world, everyone wants the comfort of preferences. With it comes the preference to personalize their devices too. This can be in the form of dark/light mode, wallpaper, ringtone. So do they get the flexibility of adjusting font size suitable to their eyes too? Yes, they get it!
Dynamic font sizes make an app readable without much effort. We can implement dynamic font sizes using (Attributes Inspector → Font → Text Styles). Same illustrated below:
a. Dynamic Font Setting for
UILabel
Note: Don't forget to select the Dynamic Type
checkmark as shown in the last step above. Without it, font scaling will not work.
Text Style works perfectly fine with UILabel
, UITextField
, and UITextView
.
b. Left side is without Text Style and right side is with Text Style
As you can see above, the UIButton
title did not change merely by this setting. This is because UIButton
does not have the option for enabling Automatically Adjusts Font
inside attributes inspector.
c. Attributes Inspector for
UIButton
So to make the user experience consistent, we handle the button just by one extra step:
button.titleLabel?.adjustsFontForContentSizeCategory = true
Though it works, adjusting the above property manually for each button is not the ideal way to handle it. Let's make an extension to manage this easily throughout all view controllers.
extension UIButton {
func setDynamicFontSize() {
NotificationCenter.default.addObserver(self, selector: #selector(setButtonDynamicFontSize),
name: UIContentSizeCategory.didChangeNotification,
object: nil)
}
@objc func setButtonDynamicFontSize() {
Common.setButtonTextSizeDynamic(button: self, textStyle: .callout)
}
}
class Common {
class func setButtonTextSizeDynamic(button: UIButton, textStyle: UIFont.TextStyle) {
button.titleLabel?.font = UIFont.preferredFont(forTextStyle: textStyle)
button.titleLabel?.adjustsFontForContentSizeCategory = true
}
}
Finally, to see the magic, you need to call setDynamicFontSize()
.
override func awakeFromNib() {
super.awakeFromNib()
openButton.setDynamicFontSize()
}
This method can either be called from the View Controller’s viewDidLoad()
or if we are using a UITableViewCell
, then from the cell’s awakeFromNib()
. Currently, we are calling it from the latter. Here is the demo for it:
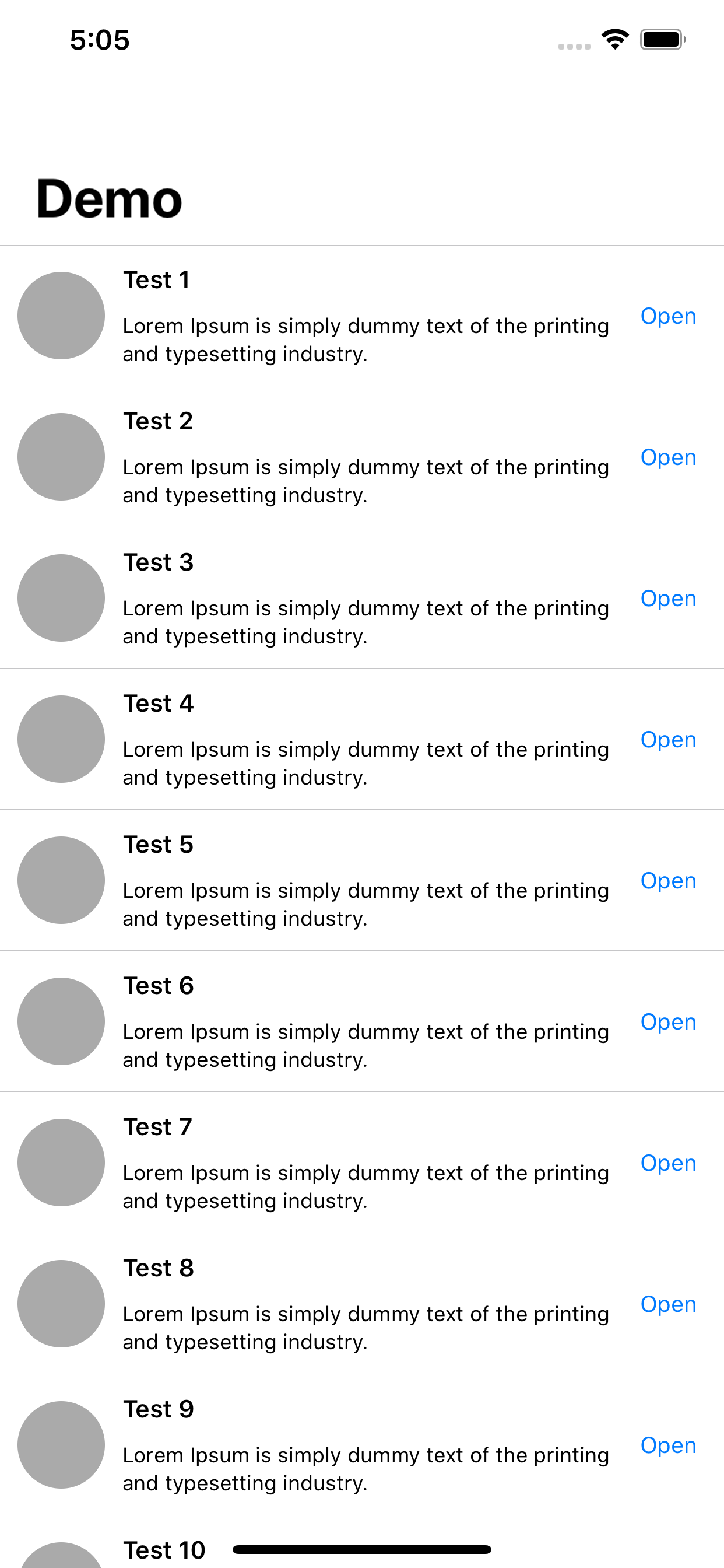
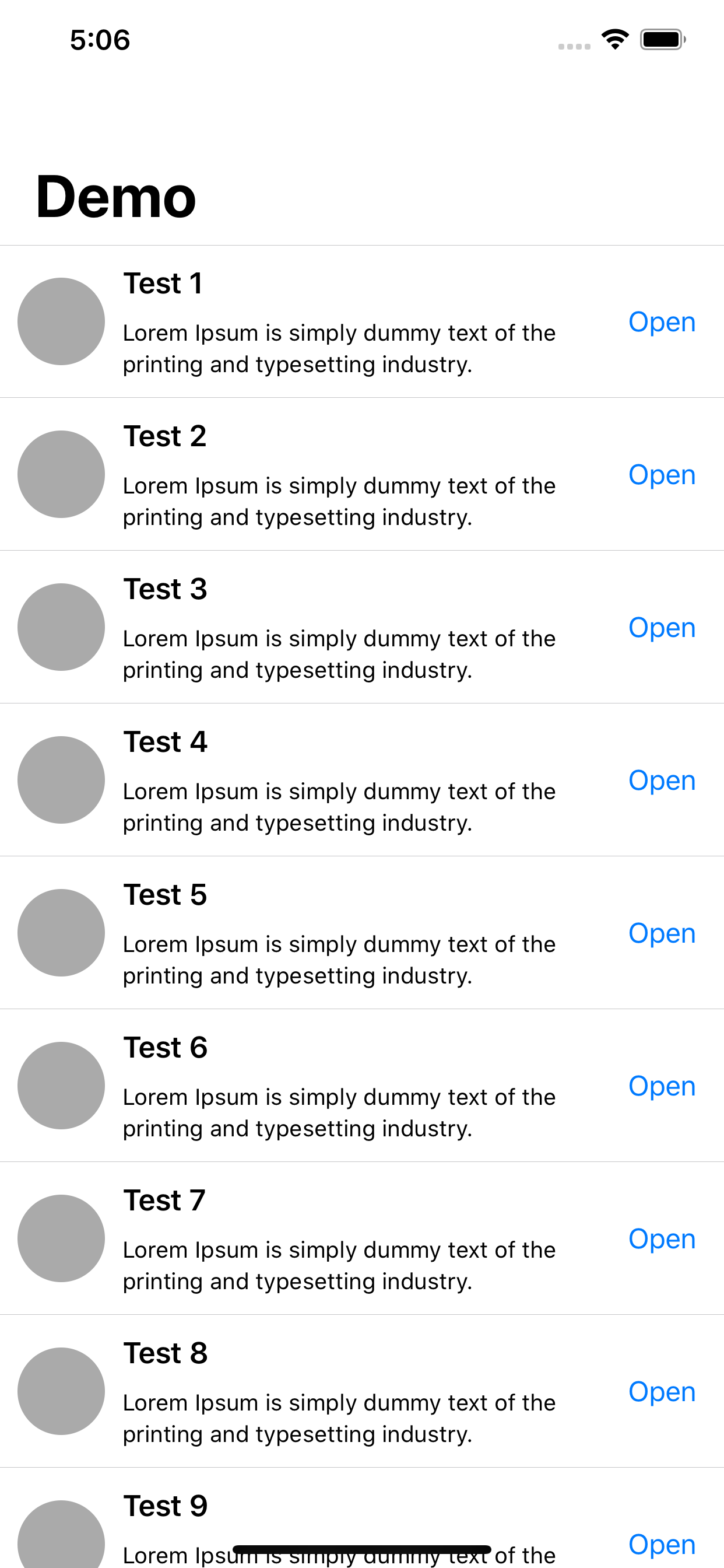
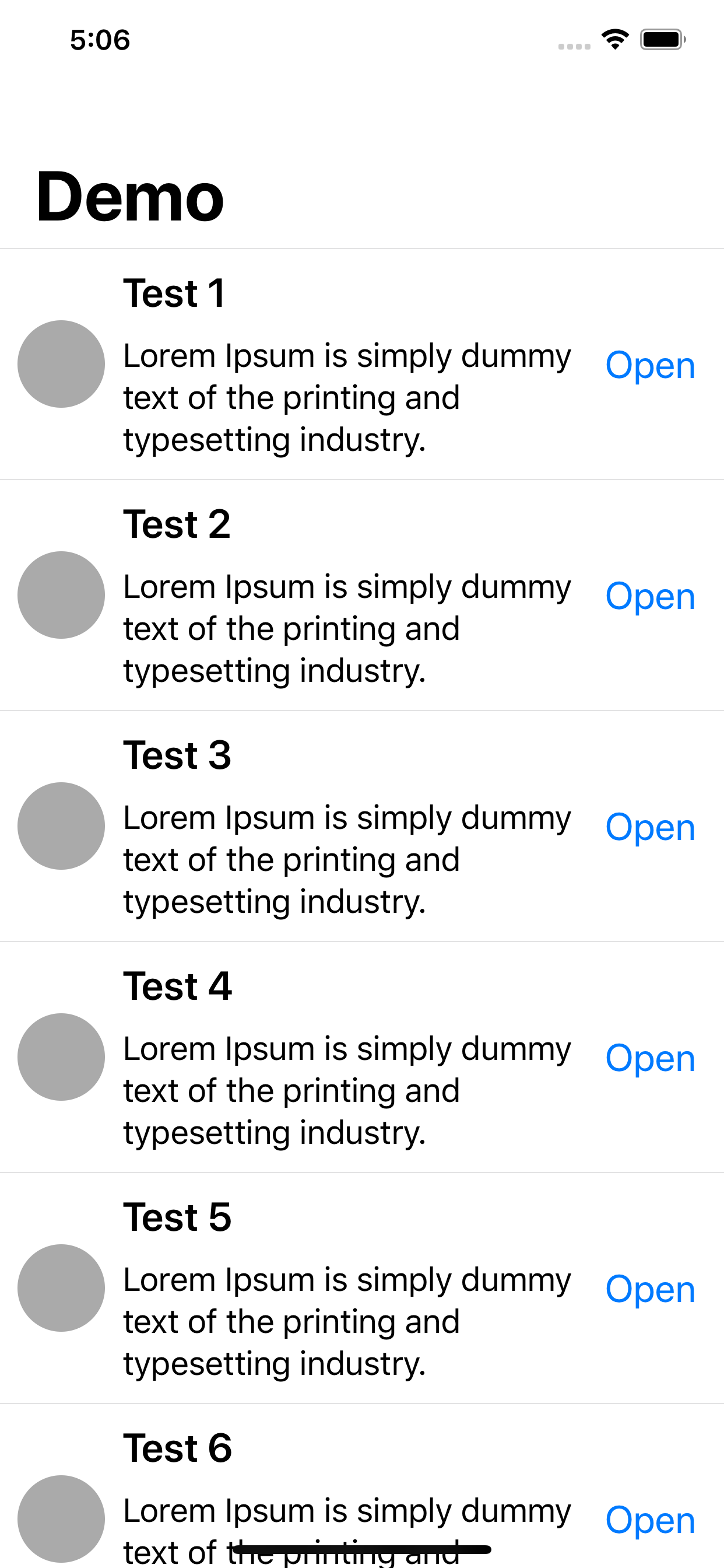
Note: This functionality is also supported by the latest version of Swift (Swift 5, at the time of writing this blog).
You can download the sample project from here. It is done in Xcode 11.2.1 (using Swift 5 and Storyboard).