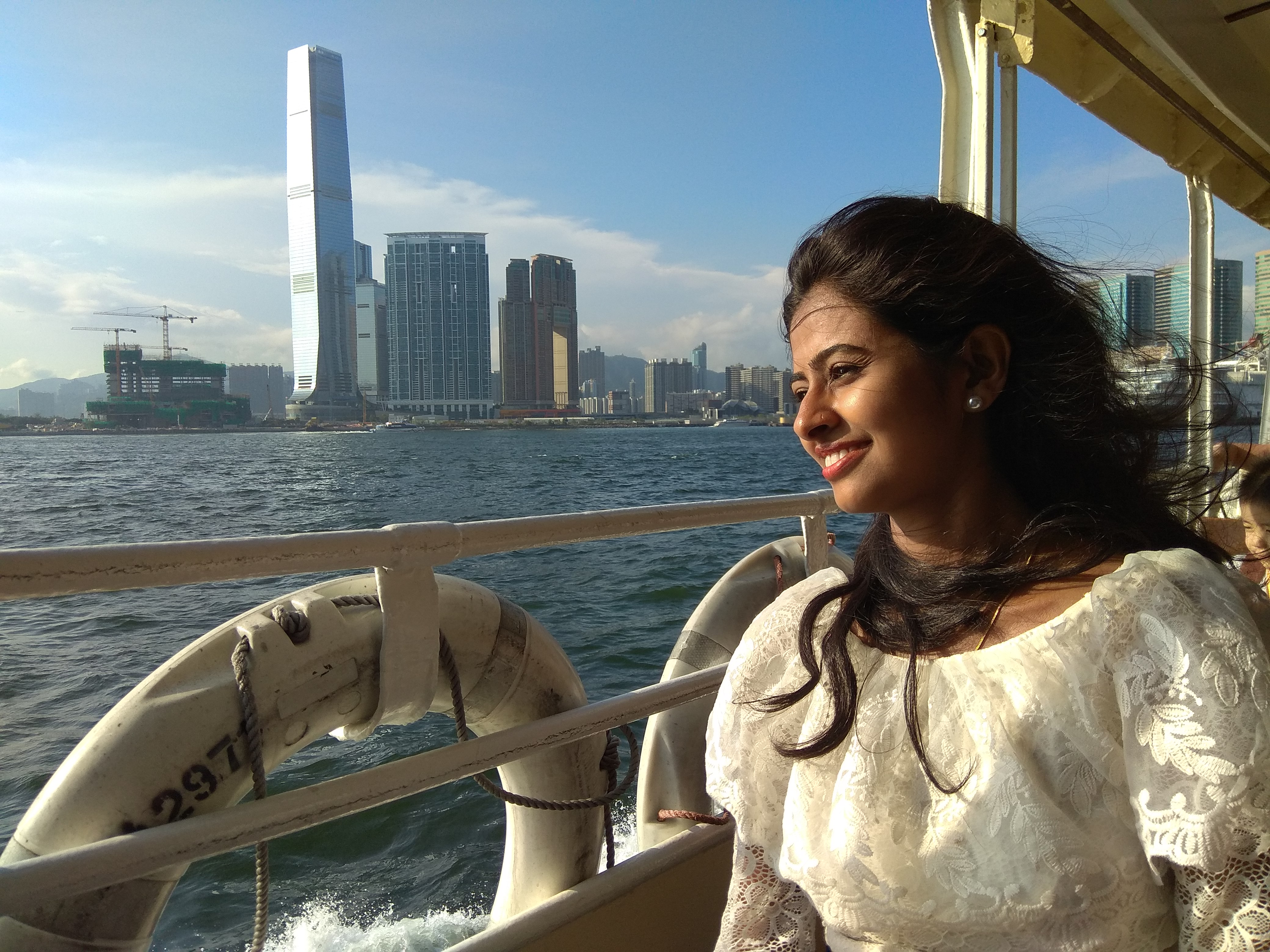
Namrata Ukirde
I am a Ruby on Rails full stack developer at Kiprosh.com. I am passionate about learning new things. I love to write poems.
Kiprosh
is now part of
LawLytics
In this article we are going to have look at some methods of Ember.computed class which helps you to refactor code or write more readable code. And most importantly if ember provides its own methods then why to write code with number of lines to do same thing. Ember.computed class documentation: Ember.computed getEach: This method returns all the values for attributes passed as argument. var names = [] this.get('model').forEach((user)=>{ names.push(user.get('name')); }); This can be simply done as: this.get('model').getEach('name'); It will return array of names. sum: This method
Ember uses the Handlebars templating library for your app's user interface. Handlebars templates contain static HTML and dynamic content. It uses data-bindings for dynamic contents. For more details you can check ember documentation: Handlebar Documentation Helpers: Helpers are functions that are designed to help you control the way your data is rendered by the template in the browser for the client to view. Helpers helps you to add additional functionality to your templates beyond what is included out-of-the-box in Ember. Helpers are most useful to transform raw values from models and components into a format more appropriate for your users.
As software developer most important thing for us is to understand debugging. When things go wrong with code, we need to be able to find the bug quickly and solve it. Today we are going to have look at some important thing regarding ember app debugging. These are small things but can help you if you are new with ember and trying to debug some error in your ember application. Ember Inspector: It is a browser extension available for Chrome and Firefox. This is helpful tool for debugging ember app. You can simply add it from webstore. You will see
While working with any application, testing plays most important role. Data is main requirement for writing proper test cases. We need different kind of data to test complex features and here factories plays important role. Data factory is blueprint that allows us to create an object, or a collection of objects, with predefined set of values. Factories makes it easy for us to define different kind of data. In rails or ember we create model object to write test cases. In rails many of us use FactoryGirl to create such test data using factories. Same way ember has FactoryGuy. Ember's
Many of us have already heard about PaperTrail gem and its use for versioning of model. I found it very useful for purpose of debugging. Lets have a quick look at exactly what is PapertTrail gem and how we can use it for purpose of debugging. PaperTrail and Versions: When we add papertrail gem in our rails app and run migration for same at that time it creates Version table for us in our DB. When we use it in particular model and perform any kind create, update operation on it then each time it creates associated versions record for
In rails one of the way to execute raw query is use of ActiveRecord::Base.connection.execute We are going to see if we use this option to execute raw query and we are working with pg database then what result it provides and what operations can be performed on that result. results = ActiveRecord::Base.connection.execute('select * from purposes') It returns PG::Result object : #<PG::Result:0x007fd5a09686d8 status=PGRES_TUPLES_OK ntuples=3 nfields=5 cmd_tuples=3> We can verify it simply using .class as we normally do in ruby. results.class => PG::Result This
Rspec has great feature and that is trait. In rspec we create factory for each class which provides the simplest set of attributes necessary to create an instance of that class. Many times we need some attributes which we do not want to add in original factory and at the same time we do not want to repeat it. In such scenario rspec trait is good option. For few attributes we need different values and we want to use it at multiple places can be another reason to use it. Without Trait: FactoryGirl.define do factory :package do description FFaker:
Many times App's rate and reviews decides app's success. Reviews by user's are important part of app and decides what are good things about our app and what improvements are needed. Now a days its common to provide Rate & Review button or link that will redirect to app store or play store. If we have cordova app then we can simply do it in following way using js. We can identify whether device is android or ios and accordingly open it in window. $('#rate-app').on('click', function(e) { if (iosDevice) { window.open('https://itunes.apple.com/us/app/
Sometimes tests can be hard to debug, especially when they’re running on a remote machine.This can be done with ssh enabled build with CircleCi using Debug via SSH option. SSH can be enabled for already running build or with rebuild option. To enable SSH access for a running build, go to the ‘Debug via SSH’ tab and click the ‘Enable SSH for this build’ button. To start a fresh build with SSH enabled, for example if you want to debug a build that has already finished, click the ‘with ssh’ button alongside ‘Rebuild’: It provides key such as
Let's assume we have route in our application: app/router.js Router.map(function() { this.route('category', { path: '/category/:id' }); } This route has dynamic segment. Whenever application will be loaded it will create url as '/category/1' , 'category/2' etc. What is Dynamic segment? A dynamic segment is a section of the path for a route which will change based on the content of a page. Dynamic segments are made up of a : followed by an identifier in path. If user navigates to 'category/5' then it will have category_id '5' to load correct category. Dynamic Segment