In the previous article of the React Design Patterns Basic to Advance
series, we became familiar with the Basic Design Patterns which are widely used in React. In this article, we will learn about the various Advanced Design Patterns in React.
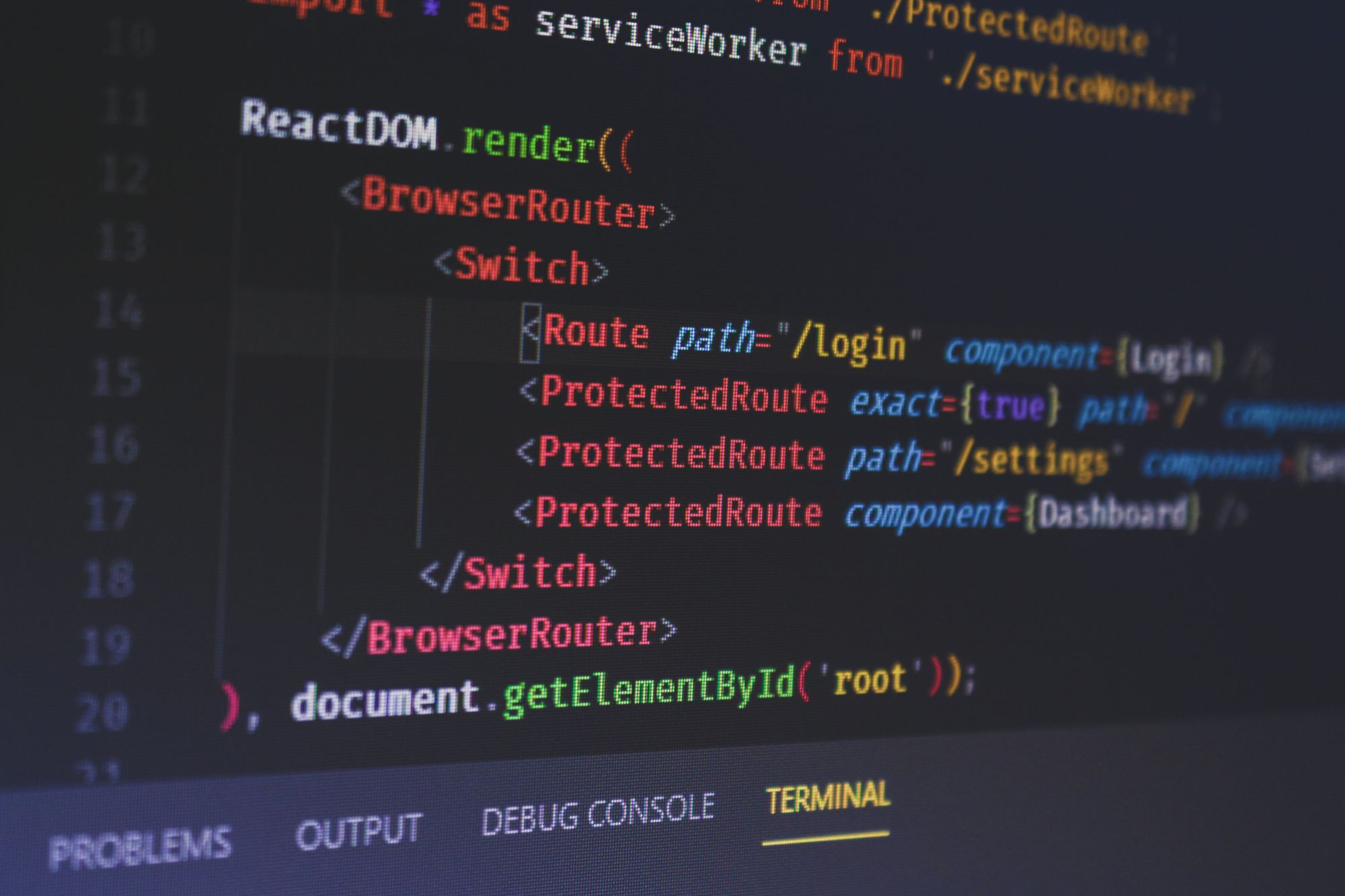
Advanced Design Patterns
-
Custom Hooks
In previous versions of React, even before v16.8, whenever we have to use state in our component and deal with lifecycle methods, we have to use Class Components. As in those versions only Class Components were capable of holding states. Function components were considered as
dumb
components. But after the introduction of Hooks, we can use state in our Function components.
Hooks are the new addition in React v16.8.
Hooks are functions that let you
hook into
React state and lifecycle features from Function components. Hooks don't work inside class components, so to use hooks we have to use React without classes (Class Components).
Why and When to use Hooks?
React doesn't offer a way toattach
reusable behavior to Component (e.g. connecting to redux-store). There are other patterns like Render props & HOC's which try to solve this problem. But the use of these patterns to solve the issue of sharing stateful logic requires the restructuring of existing components which can be cumbersome and make code harder to follow. This is where hooks come into the picture.With hooks, we can extract stateful logic from a component so it can be tested independently and reused in other components.
Hooks allow you to reuse stateful logic without changing your component hierarchy.
Refer to the example here :
Suppose we have to deal with localStorage throughout our app, so instead of accessing localStorage directly at every component we can extract that reusable logic to hook and can create a custom hook as
useLocalStorage
. And whenever we need to update or store the new value in the localStorage we can use thisuseLocalStorage
hook. -
Compound Components
The Compound component is a pattern in which components are used together such that they share an implicit state that lets them communicate with each other in the background.
Think of Compound components like the
<select>
and<option>
elements in HTML. Apart they don’t do too much, but together they allow you to create a complete experience. —Kent C. DoddsThe main idea behind compound components is that we are having two or more components that work together to accomplish a feature. Typically with this pattern, one component serves as a parent while others are the children. If we try to use one component without another then it won't even work.
Refer to the example here :
<StepWizard>
is the parent component while other form components are the child components. So here<StepWizard>
is maintaining state, current active form, and the data submitted by forms. Together it's creating a complete multi-step form experience. -
Controlled Props
In HTML form, form elements such as input, select, text area maintain their states and update it based on user input, which is termed as Uncontrolled Components. In React, the state is kept in the state property of the component and can only be updated with the state updater function. With the help of this control props pattern, we can allow users to manage the internal state of the component from outside.
Refer to the example here :
We can even mix the
Controlled
andUn-Controlled
behavior into one, such that it accepts the initial value as a prop and puts it in a state. It then reacts to props update through callback handler. -
State Reducer Pattern (useReducer)
useReducer
is one of the additional hooks, shipped with React v16.8. It's an alternative touseState
. It helps to manage the complex state in React applications. When it is combined with other hooks likeuseContext
then it can be a good alternative to other third-party state management libraries likeRedux
.
How useReducer works?
It accepts a reducer of type
(state,action)=> newState
as a first parameter and initial state as the second.
It returns an array that holds the current state value anddispatch
function to which we can pass action and later invoke. Thedispatch
function accepts an object that represents the type of action to be executed. The action is specified in the reducer function which in turn passed touseReducer
. The reducer function will then return the updated state.
Refer to the example here :
-
State Initializer pattern
Initialization means to set the value of something. By this definition and with the help of this pattern, any user can use the custom hook created and can set it to the default initial value. One important thing which is to be noted here is that this pattern doesn't give full control over setting the value of state every single time. It mostly sets the initial state within custom hook and reset state to initial default.
Refer to the example here :
To allow the user to reset the hook to the initial default value, we need to expose a callback that the user can use to reset the hook to the default value provided. In the above example, we are returning a
reset
callback which will reset the hook to the default value provided at the time of initialization.
These are the most widely used Advanced design patterns in all React application development. I hope this series of Design Patterns used in React will help you to wisely select the design pattern that is best applicable to your application requirement.
Thank you for reading!