In one of our C# applications, we recently came across a requirement to add a watermark to a pdf document. In this article, we are going to look at how we can achieve this using iText7
and another alternate way to easily do this using KiproshWatermarkingPDF
Before iText7
, there was a library iTextSharp
in C# which was used for doing operations like merging pdf, creating a page, and adding it to the pdf. But it is deprecated now. iText introduced iText7
which is a powerful PDF Toolkit.
Compared to other libraries, iText7
has an easy implementation, good documentation, and also it is open-source.
How to use iText7
to implement watermark in PDF document?
Here is the link to the utility service code base that can be utilised to implement the watermark on pdf. You can follow along to implement it in your application and, also feel free to modify it as per your requirement
In above-shared utility service code, WatermarkPdf
function adds the watermark to all the PDF pages.
But is there any easier way to achieve it with fewer lines of code? Yes. Let's see how to implement it.
How to use KiproshWatermarkingPDF
to implement watermark in PDF document?
KiproshWatermarkingPDF
is a library which internally uses iText7
to implement text/image watermark to pdf documents.
This service has different overloads which can be utilized as per requirements. Below is the list of parameters used in the service.
sourceData | pdf document as byte[] |
watermarkImgPath | watermarking image path |
watermarkText | text for watermarking |
textFont | name of font or its location on file |
fontSize | font size of watermark text |
opacity | opacity of watermark text/image |
x | x co-ordinate for placing watermark text |
y | y co-ordinate for placing watermark text |
textAlign | horizontal alignment about the specified point |
vertAlign | vertical alignment about the specified point |
radAngle | the angle of rotation applied to the text, in radians |
Here input and output parameter for pdf service is byte[]
. Stream can be used to convert respective file to byte[]
that way this service will be more efficient. In this implementation, memory stream and iText7
classes are utilized to implement watermarking.
To implement text watermark:
byte[] WatermarkPdfWithText(byte[] sourceData, string watermarkText, float? opacity)
To implement image watermark:
byte[] WatermarkPdfWithImage(byte[] sourceData, string watermarkImgPath, float? opacity)
How to use this service?
To use the service, first include the Shared.Utility
namespace to get the PDFUtility
class and call the required methods on it.
Let's take a sample PDF and now watermark it with an image. Here we will use the WatermarkPdfWithImage
method to put the watermark image on the PDF.
using Shared.Utility;
using System.IO;
namespace PdfApplication
{
class Program
{
static void Main(string[] args)
{
byte[] pdf = File.ReadAllBytes("pdfPath");
string img = "imagePath";
byte[] wbytes = PDFUtility.WatermarkPdfWithImage(pdf, img, 0.2f);
File.WriteAllBytes("watermarked.pdf", wbytes);
}
}
}
Here is the watermarked PDF
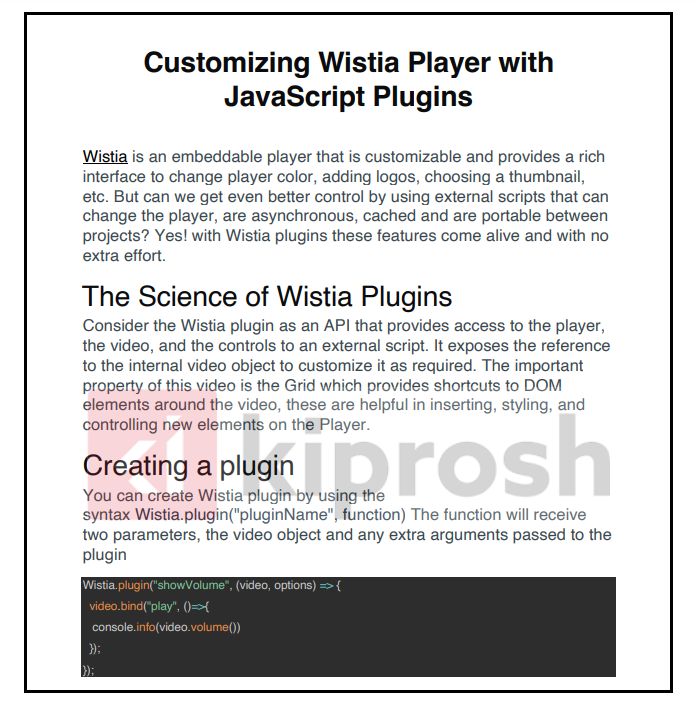
In this way, you can easily add a watermark in PDF documents. I hope this article will be helpful to implement watermarking using the iText7
and KiproshWatermarkingPDF
library in C#